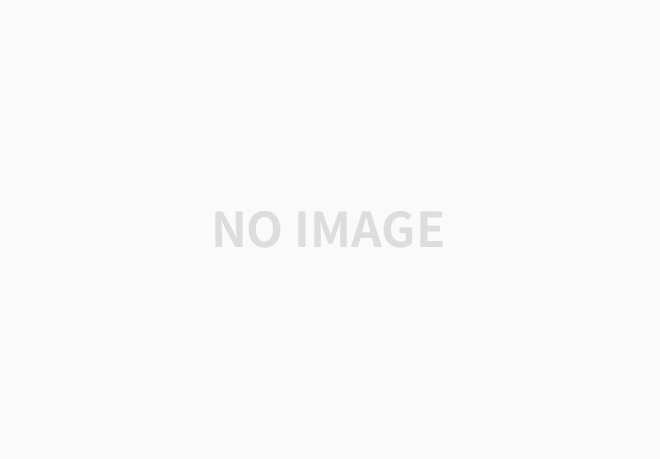
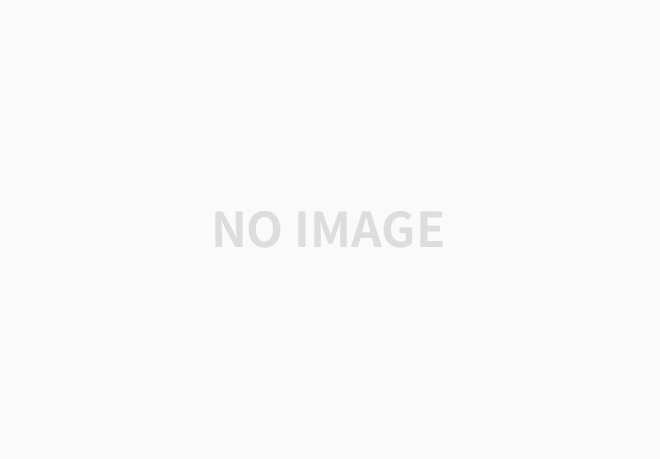
https://www.acmicpc.net/problem/10845
10845번: 큐
첫째 줄에 주어지는 명령의 수 N (1 ≤ N ≤ 10,000)이 주어진다. 둘째 줄부터 N개의 줄에는 명령이 하나씩 주어진다. 주어지는 정수는 1보다 크거나 같고, 100,000보다 작거나 같다. 문제에 나와있지
www.acmicpc.net
#
# push X: 정수 X를 큐에 넣는 연산이다.
# pop: 큐에서 가장 앞에 있는 정수를 빼고, 그 수를 출력한다. 만약 큐에 들어있는 정수가 없는 경우에는 -1을 출력한다.
# size: 큐에 들어있는 정수의 개수를 출력한다.
# empty: 큐가 비어있으면 1, 아니면 0을 출력한다.
# front: 큐의 가장 앞에 있는 정수를 출력한다. 만약 큐에 들어있는 정수가 없는 경우에는 -1을 출력한다.
# back: 큐의 가장 뒤에 있는 정수를 출력한다. 만약 큐에 들어있는 정수가 없는 경우에는 -1을 출력한다.
def push(list, a) :
list.append(a)
return list
def pop (list) :
if not list:
print(-1)
else :
print(list[0])
del(list[0])
return list
def size(list) :
return len(list)
def empty(list) :
if not list:
return 1
else :
return 0
def front(list) :
if not list:
return -1
else :
return list[0]
def back(list) :
if not list:
return -1
else :
return list[-1]
i = int(input())
list = []
for _ in range(i) :
cmd = input()
cmd = cmd.split(' ')
if cmd[0] == 'pop':
pop(list)
elif cmd[0] == 'push':
push(list, int(cmd[1]))
elif cmd[0] == 'front':
print(front(list))
elif cmd[0] == 'back':
print(back(list))
elif cmd[0] == 'empty':
print(empty(list))
elif cmd[0] == 'size':
print(size(list))
-> 이 방식으로 제출하면 채점시 시간 초과 오류가 뜬다
# 시간초과를 해결하기 위한 방안
0. switch case 교체
파이썬에서는 지원되지 않는 구문이므로 포기
1. 함수 삭제
사실 해당 내용에서의 함수는 큰 의미가 없어, 없어도 크게 영향을 받지 않음
2. input 변경
sys.stdin import
# 변경후
from sys import stdin
list = []
for _ in range(int(stdin.readline())) :
cmd = stdin.readline().split()
if cmd[0] == 'pop':
if list : print(list.pop(0))
else : print (-1)
elif cmd[0] == 'push':
list.append(cmd[1])
elif cmd[0] == 'front':
if not list: print(-1)
else : print(list[0])
elif cmd[0] == 'back':
if not list: print(-1)
else : print(list[-1])
elif cmd[0] == 'empty':
if not list: print(1)
else : print(0)
elif cmd[0] == 'size':
print(len(list))
'Python > 코딩 연습' 카테고리의 다른 글
Python / 알고리즘] 백준 #4513 (0) | 2021.10.23 |
---|---|
Python / 알고리즘] 백준 #1002 터렛 문제 (0) | 2021.10.23 |
Python] 파이썬 프로그래밍의 이해(교보문고) 4장 연습문제 (1) | 2019.02.23 |
파이썬(Python)] 시작 (0) | 2019.02.10 |